Taming the Beast: Secure Coding Best Practices in C
- Jason Gravelle
- May 1
- 3 min read
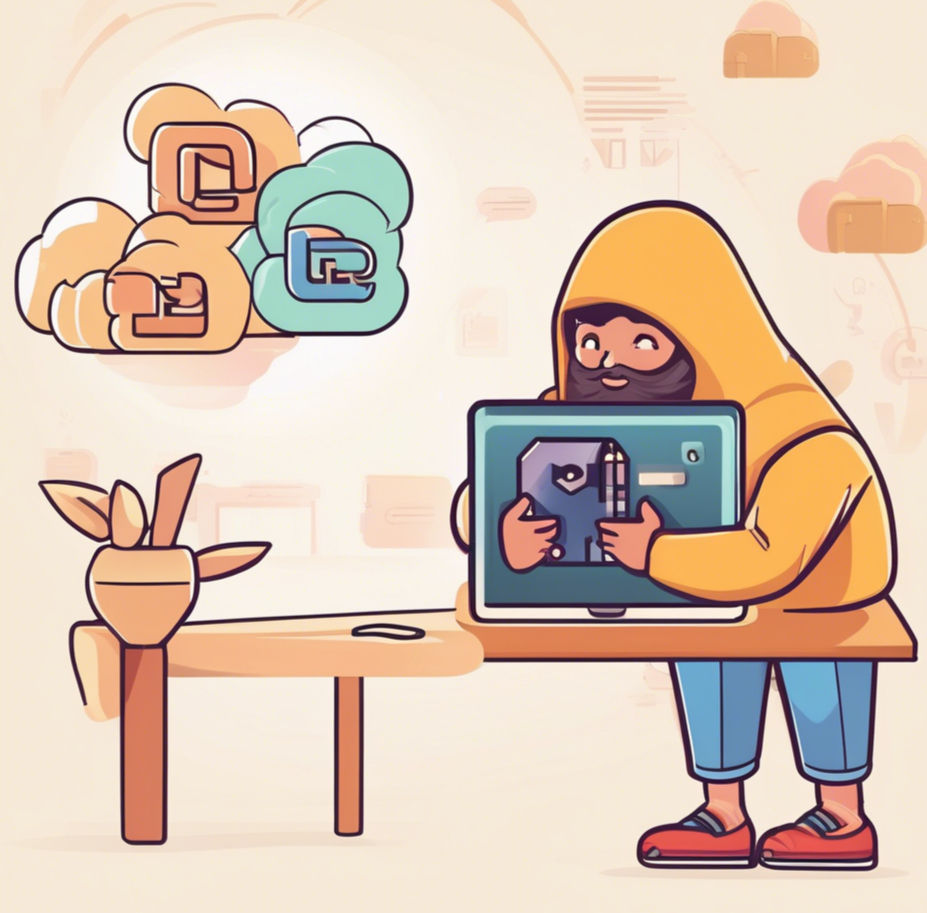
The C programming language remains a cornerstone of modern computing. Its power, performance, and low-level control make it indispensable for operating systems, embedded systems, high-performance computing, and countless other applications. However, this power comes with significant responsibility. Unlike many modern languages, C offers fewer built-in safety nets, placing the onus squarely on the developer to write secure and robust code.
In 2025, with cyber threats constantly evolving, adhering to secure coding practices in C isn't just good practice – it's essential. Neglecting these can lead to serious vulnerabilities like buffer overflows, memory corruption, and injection attacks. Here are some crucial considerations and best practices every C developer should employ:
The Double-Edged Sword: Why C Needs Extra Care
C gives developers direct memory access and pointer manipulation capabilities. While powerful, this means common programming errors can have severe security consequences:
Manual Memory Management: Incorrectly managing memory allocation (malloc, free) and usage is the source of many critical C vulnerabilities (buffer overflows, use-after-free, double frees, memory leaks).
Pointers: Improper pointer arithmetic or dereferencing invalid pointers can lead to crashes or exploitable conditions.
Integer Issues: Integer overflows or underflows, especially when calculating buffer sizes or array indices, can lead to dangerous memory errors.
String Handling: Standard C library functions for string manipulation (like strcpy, strcat, sprintf) are notoriously unsafe if buffer sizes aren't meticulously managed.
Essential Secure C Practices
Master Memory Management (The Cardinal Rule):
Prevent Buffer Overflows: Always check buffer boundaries before writing. Use size-limited functions like strncpy(), strncat(), and especially snprintf() instead of their unsafe counterparts (strcpy(), strcat(), sprintf()). Be mindful that strncpy might not null-terminate if the buffer fills completely. Explicitly check array indices and loop bounds.
Allocate/Deallocate Carefully: Always check the return value of malloc() and calloc() for NULL. Ensure every malloc/calloc has a corresponding free(). Avoid double-freeing memory (set pointers to NULL immediately after freeing).
Avoid Use-After-Free: Do not use pointers after the memory they reference has been free()d.
Validate All External Inputs Rigorously:
Treat any data coming from outside your program's trust boundary (user input, network data, files, environment variables) as potentially hostile.
Validate input for type, length, format, and range before using it, especially before using it to determine buffer sizes or control program flow. Sanitize input where appropriate.
Beware Integer Overflows/Underflows:
Integer overflows (when a calculation exceeds the maximum value for its type) can wrap around and become small numbers, often leading to insufficient memory allocation followed by a buffer overflow.
Be especially careful with integer arithmetic used for size calculations or array indexing. Check for potential overflow conditions before performing critical operations. Consider using safer integer libraries if available for your project.
Handle Strings Safely:
Strongly prefer bounded functions like snprintf() which prevent buffer overflows and ensure null termination.
If using strncpy() or strncat(), always manually ensure null termination within the buffer bounds. Track string lengths explicitly whenever possible.
Use Pointers Judiciously and Carefully:
Initialize all pointers before use (often to NULL).
Minimize complex pointer arithmetic.
Be extremely cautious with pointer casting, ensuring type compatibility. Check pointers against NULL before dereferencing.
Secure Format String Usage:
Never use user-controlled strings directly as the format specifier in functions like printf(), sprintf(), syslog(), etc. This can lead to format string vulnerabilities allowing attackers to read memory or cause crashes. Always use static format strings (e.g., printf("%s", user_input); NOT printf(user_input);).
Check Return Codes Religiously:
Many C library and system calls return values indicating success or failure (e.g., malloc, file operations, network calls). Always check these return codes and handle potential errors gracefully and securely, without leaking sensitive information.
Leverage Your Tooling:
Compiler Warnings: Enable the highest warning levels (-Wall -Wextra -pedantic or similar for GCC/Clang) and treat warnings as errors (-Werror). These often flag potentially unsafe code constructs.
Static Analysis (SAST): Use static analysis tools specifically designed for C (like Clang Static Analyzer, Cppcheck, PVS-Studio, commercial tools) to automatically find potential bugs and vulnerabilities in your source code.
Dynamic Analysis: Use memory debugging tools (like Valgrind, AddressSanitizer - ASan, MemorySanitizer - MSan) during testing to detect memory errors at runtime.
Beyond Code: Process Matters
Secure coding practices should be part of a broader Secure Software Development Lifecycle (SSDLC). Include threat modeling during design, conduct security-focused code reviews (paying special attention to the C-specific risks mentioned above), and employ testing strategies like fuzzing, which is highly effective at finding vulnerabilities in C code.
Programming in C offers unparalleled control and performance, but it demands discipline and a constant focus on security. Manual memory management and pointer arithmetic are powerful but unforgiving. By diligently applying secure coding practices, rigorously validating input, leveraging modern tooling, and fostering a security-aware development culture, you can mitigate the inherent risks and build robust, reliable, and significantly more secure C applications. It's a challenge, but one that's essential to meet in today's security landscape.
Comments